💡 สวัสดีจ้าเพื่อน ๆ วันนี้แอดจะพาเพื่อน ๆ มารู้จักกับ Operator จาก JavaScript ที่จะช่วยให้เพื่อน ๆ เข้าถึงข้อมูลใน Object ได้ง่ายมากขึ้น !!
.
🌈 และเจ้านี่คือ...Optional chaining (?.) นั่นเองจ้า จะเป็นยังไง มีรายละเอียด และวิธีการใช้งานยังไง ไปติดตามกันได้ในโพสต์นี้เลยจ้า ~~
.
✨ Optional chaining (?.) - เป็นตัวดำเนินการที่ทำให้เราสามารถอ่านค่าใน Object ที่ซ้อนกันหลาย ๆ ชั้นได้ง่ายมากขึ้น เขียนง่าย และทำให้โค้ดสั้นลงนั่นเอง
.
จริง ๆ แล้วมันก็เหมือนเราใช้ เครื่องหมายจุด (.) นั่นแหละ แต่ความพิเศษของมันก็คือถ้าในกรณีไม่มีค่าใน Object หรือ Function มันจะ Return เป็น Undefined แทน Error
.
👨💻 Syntax
.
obj.val?.prop
obj.val?.[expr]
obj.arr?.[index]
obj.func?.(args)
.
📑 วิธีการใช้งาน
.
❤️ ตัวอย่าง 1 : ใช้เข้าถึงข้อมูลใน Object
let customer = {
name: "Mew",
details: {
age: 19,
location: "Ladprao",
city: "bangkok"
}
};
let customerCity = customer.details?.city;
console.log(customerCity);
//output => bangkok
.
❤️ ตัวอย่าง 2 : ใช้กับ Nullish Coalescing
let customer = {
name: "Mew",
details: {
age: 19,
location: "Ladprao",
city: "bangkok"
}
};
const customerName = customer?.name ?? "Unknown customer name";
console.log(customerName); //output => Mew
.
❤️ ตัวอย่าง 3 : ใช้กับ Array
const obj1 = {
arr1:[45,25,14,7,1],
obj2: {
arr2:[15,112,9,10,11]
}
}
console.log(obj1?.obj2?.arr2[1]); // output => 112
console.log(obj1?.arr1[5]); // output => undefined
.
📑 Source : https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Optional_chaining
.
borntoDev - 🦖 สร้างการเรียนรู้ที่ดีสำหรับสายไอทีในทุกวัน
#javascript #optionalchaining #BorntoDev
同時也有16部Youtube影片,追蹤數超過14萬的網紅アスキー,也在其Youtube影片中提到,The Solid Knitting Machine is a new, innovative take on the classic craft of knitting. This machine is set to be improved and will soon be possible to...
「return object object」的推薦目錄:
return object object 在 BorntoDev Facebook 的最讚貼文
💡 หลาย ๆ คนที่เขียน Python มาสักพักแล้ว หรือบางคนเพิ่งเริ่มเขียน อาจจะยังไม่รู้จักการใช้งาน Iterator และ Iterable กันสักเท่าไหร่ หรือบางคนอาจจะใช้งานอยู่แล้ว แต่ยังไม่รู้ความหมายของมัน…
.
🔥 วันนี้แอดมาสรุปการใช้งาน Iterator และ Iterable มาให้เพื่อน ๆ อ่าน จะได้กระจ่างกันเลยว่าทั้งสองเนี่ยมันคืออะไร ใช้งานยังไง ถ้าพร้อมแล้วไปอ่านกันเลยยยยย!!
.
Iterable - Object ที่สามารถวนซ้ำได้ (List, Tuple, และ Strings ก็เป็น Iterable)
Iterator - ตัวที่ใช้วนซ้ำ
.
ประกอบด้วย Methods ดังนี้
🔹 __iter __ () - ใช้สร้าง Iterator เพื่อวนซ้ำใน Iterable
🔹 __next __ () - ใช้ดึงข้อมูลออกจาก Iterable
.
⚙️ การใช้งาน
iter() จะทำการสร้าง Iterator เพื่อกำหนดการวนซ้ำให้กับ Iterable จากนั้น และ next() จะดึงข้อมูลใน Iterable ออกมาตามลำดับการวนซ้ำนั่นเอง
.
👨💻 ตัวอย่าง1 : ดึงค่าใน Iterable ออกมาตามลำดับ Index
fruit = ["Apple", "papaya", "banana", "orange"]
iterator = iter(fruit)
print(next(iterator))
print(next(iterator))
print(next(iterator))
print(next(iterator))
.
หากเรียกใช้ 'next(iterator_obj)' อีกครั้ง มันจะ Return 'StopIteration' ออกมา เพราะค่าถูกดึงออกมาครบแล้วนั่นเอง
.
📑 ผลลัพธ์
Apple
papaya
banana
orange
.
👨💻 ตัวอย่าง2 : ตรวจสอบค่าใน Object ที่กำหนดว่าเป็น Iterable หรือไม่
def iterable(y):
try:
iter(y)
return True
except TypeError:
return False
arr = [34, [24, 35], (44, 112), {"Prayut":250}]
for i in arr:
print(i, " is iterable : ", iterable(i))
.
📑 ผลลัพธ์
34 is iterable : False
[24, 35] is iterable : True
(44, 112) is iterable : True
{'Prayut': 250} is iterable : True
จะเห็นว่า 34 ไม่ได้เป็น Iterable นั่นเอง
.
💥 Source : https://www.geeksforgeeks.org/python-difference-iterable-iterator/
.
borntoDev - 🦖 สร้างการเรียนรู้ที่ดีสำหรับสายไอทีในทุกวัน
return object object 在 BorntoDev Facebook 的最佳解答
🎁 วันนี้มาพบกับช่วงของดีบอกต่อกับคอร์ส TypeScript Course for Beginners 2021 จากช่อง Academind ที่จะทำให้เราเขียน Typescript เป็นภายใน 3 ชม. !!
.
📚 คลิปนี้ประกอบด้วยเนื้อหาที่จะช่วยให้เราเข้าใจ Typescript ซึ่งเล่าตั้งแต่ที่มา และยังเข้าใจความแตกต่างของ TypeScript และ JavaScript อีกด้วยนะ แถมยังเป็นการสอนแบบอธิบายโค้ดให้ดูเลย เข้าใจง่ายมากเลยคร้าบบ
.
⚡สำหรับคลิปนี้ประกอบด้วยเนื้อหาดังนี้ (อ้างอิงจาก Timestamp)
✅Getting Started
✅What is TypeScript
✅Installing & Using TypeScript
✅The Advantages of TypeScript
✅Course Outline
✅How to Get the Most out of This Course
✅Setting Up our Development Environment
✅The Course Project Setup
✅Module Introduction
✅Using Types
✅TypeScript Types vs JavaScript Types
✅Numbers, Strings and Booleans
✅Type Assignment and Type Inference
✅Object Types
✅Array Types
✅Tuples
✅Enums
✅The Any Type
✅Union Types
✅Literal Types
✅Type Aliases
✅Function Return Types and Void
✅Function Types
✅Function Types and Callbacks
✅The Unknown Type
✅The Never Type
✅Wrap Up
✅Module Introduction
✅Watch Node
✅Compiling the Entire Project
✅Include and Exclude Files
✅Setting a Compilation Target
✅Understanding TypeScript Libs
✅More Options
✅Source Maps
✅Rootdir and Outdir
✅noemit on Error
✅Strict Compilation Options
✅Code Quality Options
✅Debugging with Visual Studio Code
✅Wrap Up
.
💥ถ้าใครสนใจคอร์สนี้ กดลิงค์เข้าไปเรียนกันเลยจ้า !!! >>
https://www.youtube.com/watch?v=BwuLxPH8IDs
.
borntoDev - 🦖 สร้างการเรียนรู้ที่ดีสำหรับสายไอทีในทุกวัน
return object object 在 アスキー Youtube 的精選貼文
The Solid Knitting Machine is a new, innovative take on the classic craft of knitting. This machine is set to be improved and will soon be possible to craft and update objects based on inputted 3D data.
Knitting can be thought of as digital, where the composition of the knitted object is constructed by the organization of individual stitches. This digital essence allows us to undo, reknit, and copy using a knitting pattern. Currently, knitting is only used to make fabric or cloth, but if it can make shapes with solid centers, it could be used to make other physical, solid objects such as chairs or desks. For example, you could unravel a chair, return it to its original yarn, and update it into a desk. This is solid knitting. The Solid Knitting Machine will automate that process.
ソリッド・ニッティング・マシンは編み物を革新的に発展させる技術を持った編み機です。近い将来、入力された3Dデータに基づき中身の詰まった物体を造形する特殊な編み機になるだろう
編み物は、1目1目の集合によって全体を構成する、まさしくデジタルなものづくりです。
このデジタルな特徴により、元の状態に戻したり(undo)、そこから編み直したり(update)、編み図を用いて複製したり(copy)することができます。
編み物は現状布地をつくることにのみ使われていますが、これで中身の詰まった立体をつくることができれば、このデジタルな性質を机や椅子といった「かたいもの」にも応用できます。
たとえば、椅子をほどいて元の糸に戻し、その糸を机に編み直すといったことができるようになります。これがソリッド編みです。
このソリッド編みを自動化する機械、ソリッド・ニッティング・マシンをご紹介します。
Subscribe and see more innovative tech: https://bit.ly/3djZ5G3
Official Website: https://innouvators.com/en/
Twitter: https://twitter.com/InnoUvators
Facebook: https://www.facebook.com/InnoUvators/
Instagram: https://www.instagram.com/innouvators/
----------------------------------------------
★ムービーサイト「アスキーTV」http://ascii.jp/asciitv/
★ニュースサイト「ASCII.jp」http://ascii.jp/
★超ファンクラブ「ASCII倶楽部」http://ascii.jp/asciiclub/
----------------------------------------------
#異能vation #innovation
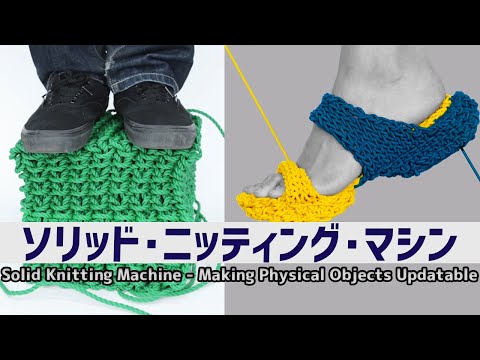
return object object 在 もちよ/ mochiyo Youtube 的最佳解答
スライムジャパンさんの「ぽっちゃりマカロン -オレオ-」
"Chubby macaron -Oreo-" by Slime Japan
0:00 〜 OP
0:45 〜 本編開始
Start of the main movie
0:52 〜 ベーススライムを触る
Touch the base slime
3:19 〜 ホウ砂調節
activate with borax
3:39 〜 DIY TIME☆ (with BGM)
4:24 〜 触り始め
begin to touch
4:32 〜 両手でガッツリ遊ぶ
play with both hands
8:11 〜 あたたたたたたいむ
mochiyo beam
8:17 〜 もこもこテクスチャーで遊ぶ
play with a fluffy texture
9:38 〜 もこもこにして容器に還元 fluff up and return to the container
9:45 〜 もこもこしゅわしゅわ
fluffy & sizzly
10:58 〜 もこもこしゅわしゅわ
fluffy & sizzly
11:50 〜 もこもこしゅわしゅわ
fluffy & sizzly
13:26 〜 もこもこしゅわしゅわ
fluffy & sizzly
クラウドクリームにクレイを混ぜて楽しむDIYスライムです
A DIY slime that I can enjoy by mixing clay with cloud cream.
まずはベースのクラウドクリームから遊んでいきます
Let's start with the base slime, cloud cream slime.
砕いたオレオみたいなチップが美味しそうです!
Chips like crushed Oreo look delicious!
すごく滑らかなテクスチャーです
It has a very smooth texture.
チャキチャキと軽快なタップ音が鳴ります♪
I heard a wonderful tapping sound♪
伸びやかでオレオアイスみたいです……美味しそう……
It's easy to stretch and looks like Oreo ice cream ...
優しいバブル音が鳴ってくれます
I heard a soft bubble sound. I was healed.
トルコアイスみたいに見えてきました!
Now it looks like Turkish ice cream!
それではもうしばし、ベーススライムの様子をお楽しみください!
Let's enjoy the video of playing with base slime for akurimot
クレイのマカロンに挟むクリーム部分です
It is a cream part sandwiched between clay macaroons.
ホウ砂水で硬くしていきます!
I will activate this with borax water!
DIYしていきます!
Let's DIY!
マカロンクレイめっちゃ可愛い!!
Fake macaroons are so cute!
クリームを挟みます!
Put the cream between the macaroons!
めちゃめちゃ可愛いいいい!!!
It's soooooooooooooooo cute!!!!!
この可愛すぎる物体を混ぜていきます!
I will mix this too cute object!
クレイがすんなり溶け込むので、マーブルが美しいです
This marble is beautiful because the clay blends in smoothly.
この色味がもう大好物です
I really love this color.
楽しみながらゆったり混ぜていきます!
I will mix it loosely while having fun!
この混ざった後の色も大好きです!
I also love the color after this mixture!
膜は薄めで柔らかくて丸いバブル音が聴けます
The film is thin and you can hear a soft and round bubble sound.
トゥンカロンとは、クリームたっぷりのマカロンです!
Chubby macaron is a macaron with plenty of cream in it!
この伸びの良さが最高です!クリーミー!
It's very easy to stretch! Creamy!
とっても膨らみやすいフラッフィーテクスチャーです
This is a fluffy texture that is very easy to swell.
膨らみやすくするために調節します!
Activate with borax to make it easier to swell!
後半はもっともこもこにするので是非お楽しみください!
I will inflate it more and more later!
ハイジの白パンよりも柔らかくてクリームっぽい感じでした!
It was softer and creamier than "Heidi's White Bread"!
すごく好きなふかふかテクスチャーでした!
It was a very favorite fluffy texture!
チーズケーキとチョコとクリームの香りでした!
It was the scent of cheesecake, chocolate and cream!
美味しそうで濃厚なあま〜い香りでした!
It was a sweet and delicious scent!
アタタタタタタタァァッッ!!
Hissatsu! Mochiyo Beam!!!!
シュワシュワ天国うううう!!
sizzly heaven I’m dying!!
このふわふわに顔から飛び込みたい
I want to dive into this fluffy.
フワッフワのシュワシュワに埋もれて眠りたい
I want to sleep buried in fluffy sizzky heaven.
このはんぺんのようなシュワシュワがたまらんのです……
This hanpen-like sizzless is the best ...
それでは引き続きASMRをお楽しみ下さい!
Please continue to enjoy ASMR!
ごちそうさまでした!
Thank you for the meal!lol
ご視聴ありがとうございました!
Thank you for watching!!!!
#スライムジャパン #slimejapan
o8@gmail.com while!
〜*〜〜*〜〜*〜〜*〜〜*〜〜*〜
サブチャンネル【もちよの研究室】はコチラ!
↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓
https://www.youtube.com/channel/UCWmSQDBSNQTX6kpFm6lYLnw
Instagram, twitter, BASE shop, メルカリはこちら!
↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓
https://linktr.ee/mochiyoslimestore
スライムの提供についてはコチラ!
↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓
https://www.instagram.com/s/aGlnaGxpZ2h0OjE3ODQ4ODU4NzU3MDI2MzA3?igshid=1hr3jy34zfnpo&story_media_id=2286885986591781571
イヤフォンやヘッドフォンをして聴いて頂くとよりいい音で楽しめるかとおもいますので、是非に😎
また、画面右上のチョンチョンチョンのとこから画質を1080pに設定して動画を見ていただけると、高画質でお楽しみ頂けます💪💪
どうもこんびんは!
もちよすらいむです🧜🏻♀️
有名なスライマーさんのスライムのレビューや、自分で作ったスライムの動画などのASMRを中心に、いろいろなジャンルの動画を上げていきたいと思います!
太古の動画や、short ver.の動画、編集実況などは全てインスタグラムのアカウントの方にあります。インスタライブでスライムを触ったりもします。
興味を持ってくだされば、是非インスタアカウントのもチェックして頂きたいです☺️
↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓ ↓
https://www.instagram.com/mochiyoslime
*大学に通いつつ資格試験の勉強もしている学生の身ですので、更新が突然途絶える可能性があります🙇♀️
*慢性鼻炎でして、呼吸音が入ってしまうことがあります🤦♀️
また、机に爪がコツンと当たる音が入ってしまうことがあります。苦手な方は、ご視聴非推奨です🙇♀️
*自室にて、マイクを使って撮影してます!ですが、多少は「サーー」というホワイトノイズが入っています。また、稀ですが実家ぐらしなので家族の出す生活音が入ってしまう可能性があります。そういったものが苦手な方にも、ご視聴非推奨です🙇♀️
*動画を見てくださりありがとうございます💕そしてこの概要を最後まで読んでくださりありがとうございます💕
是非チャンネル登録をして、これからももちよの動画をお楽しみください💁♀️
〜*〜〜*〜〜*〜〜*〜〜*〜〜*〜
#もちよすらいむ #mochiyoslime #べらちゃんのslimeしばき部屋 #slime #asmr #asmrsounds #asmrslime #スライム #音フェチ #音フェチ動画 #音フェチスライム #clayslime #slayslime #butterslime #cloudcream #thickslime #thickie #thickieslime #fluffyslime #clearslime #slusheeslime #slushieslime #fishbowlslime #crunchyslime #slimejapan #スライムジャパン #sakuraslime #さくらスライム #aisu屋さん #tiaslime #slimeogproof #ogslimeproof #aobaslime #awesomeslimeproof #awesomeslime #slimefantasies #slimefantasiesproof
#rodemslime #rodemslimeproof #mooncottonslime #slimebyktmproof
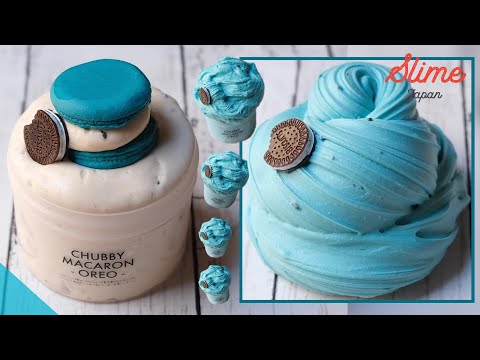
return object object 在 Shiney Youtube 的精選貼文
Watch Dogs: Legion
ซื้อเกมได้ที่ https://store.epicgames.com/legendauser/watch-dogs-legion
Watch Dogs: Legion is an action-adventure game played from a third-person perspective, and takes place within an open world setting based upon London, which can be explored either on foot - utilizing parkour moves - vehicles, or fast-travelling via the city's Underground stations. The game is composed of several missions, including those that progress the main story, liberation missions aimed at freeing the city's boroughs featured in the setting, recruitment missions for new playable characters, and various side-activities, with players able to freely pursue a mission or activity, or explore the city for secrets and collectibles. Each mission's objectives can be handled via one or several different approaches: an open-combat approach utilizing a variety of weapons; a stealth approach utilizing the environment to avoid detection and monitoring enemy patterns; or a hacking approach using any hackable object to subdue enemies with traps or distractions, while seeking out objectives via cameras and remotely accessing them. Combat includes a mixture of gun fights - involving lethal and non-lethal fire-arms - and hand-to-hand combat moves, with enemies making use of different methods depending on how the player acts against them in combat (i.e. a guard hit with a punch will use melee attacks). Players can be pursued by enemies when escaping, including hostile drones, but can lose them by utilizing hack-able environmental objects (i.e. vents) and avoiding line of sight with pursuers.
Unlike previous games in the series, Legion features the ability to use multiple characters during a playthrough, each of whom can be recruited from around the game's setting. While the player must choose a character to begin with after the story's prologue chapter, others may be recruited upon completing the initial story missions of the game from anywhere around the game's setting, which can also include those working for hostile factions. Those recruited become operatives that the player can freely switch to at any time, as well as customize with different clothing options, with each recruit-able character maintaining their own lifestyle and occupation when not active (i.e. spending time drinking at a pub). Each character that can be recruited have different traits and skills, based upon their background - a spy operative has access to a silenced pistol and can summon a special spy vehicle to travel around with, armed with rockets; a hooligan operative can summon in friends to help in a fist-fight; a builder operative can make use of large drones for heavy-lifting and a nail-gun for combat; while an "adrenaline junkie" operative can deal more damage, but risk the possibility of being knocked out/dying at any random moments. Operatives can gain experience when used by the player, which allows them to gain additional skills and abilities to improve them, with the player able to provide additional upgrades for all character by spending "tech points" - a collectible scattered around the city, which can be spent on weapon and gadget upgrades.
All potential recruits have an additional statistic, which details whether they can be recruited when approached - their thoughts on DedSec. Some recruits may not join if either they favour those that oppose them (such as a hostile faction), if the player has a character in their roster whom they hate or if DedSec did something to harm another NPC they have good relations with. If a recruit can be brought in, players will be required to complete a mission from them related to a problem they need resolving. An example of such a mission would be helping someone determine why they are being constantly spied on more frequently of late working as a vigilante in shutting down a criminal operation they seek to disrupt. Any character that can be recruited, can be killed during a playthrough by criminal gangs or law enforcement, and thus be permanently removed from the player's roster of playable characters, provided the player has the permadeath option enabled; if not, the character is merely arrested, and can be simply rescued by another operative. If the player loses all their characters from death or arrest, the game ends.
The online component of the game would be introduced through a free post-launch update in December 2020. Players can also join a team of up to four players in cooperative gameplay, sharing progression between single-player and multiplayer modes. They can also access Tactical Ops, which are co-operative missions designed for 4 players, or simply explore London together. The asymmetrical multiplayer mode Invasion would also return. The game also features a competitive multiplayer mode named "Spiderbot Arena" in which players assumes control of a gadget named spiderbot and compete against each other in free for all matches.
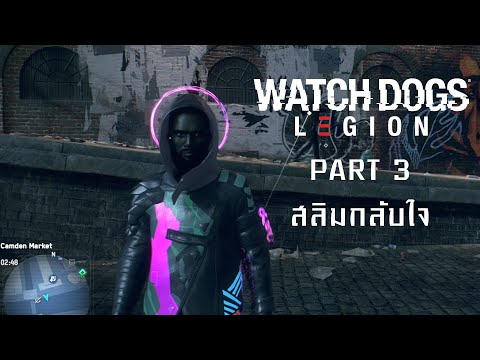